Introduction to Object-Oriented Programming (OOP)
Explore the fundamentals of Object-Oriented Programming (OOP) with our comprehensive guide. Learn key concepts and principles for modern software development.
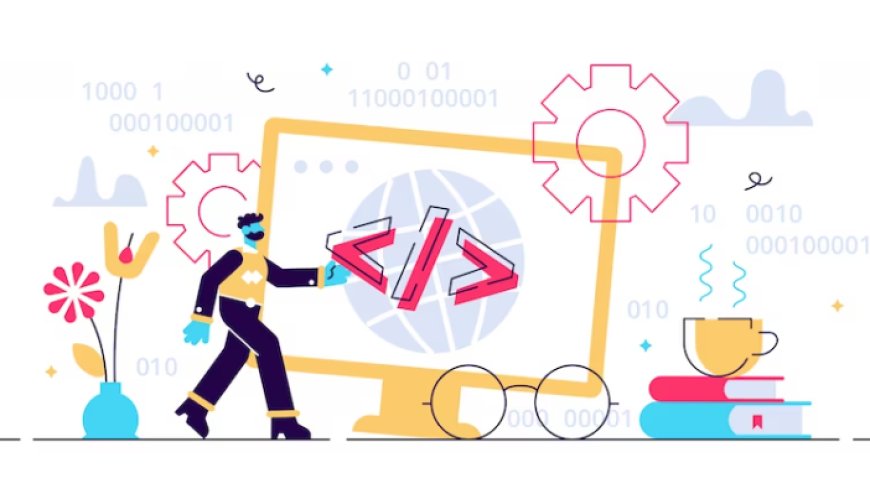
Understanding Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a foundational programming paradigm that revolutionized the way software is designed and developed. At its core, OOP centres around the concept of objects, which are self-contained units that combine data and the methods that operate on that data. This shift from traditional procedural programming, which emphasizes functions and logic, has led to significant advancements in software development.
The importance of OOP in modern programming cannot be overstated. It encourages the creation of reusable code, fostering efficiency and reducing redundancy. Objects encapsulate data and behaviour, allowing for modular, organized, and maintainable code, making it easier to solve complex problems. This modularity not only simplifies the development process but also supports scalability as applications can be extended without disrupting existing functionality.
Explain the key concepts of OOP:
Classes: Classes serve as blueprints or templates for creating objects. They define the structure and behavior of objects. Think of a class as a blueprint for a house, describing how it should be built.
Objects: Objects are instances of classes. They are tangible entities created based on the class's blueprint. An object contains data (attributes) and functions (methods) that operate on that data. For example, if the class is "Car," an object would represent a specific car with its unique characteristics.
Encapsulation: Encapsulation is a fundamental concept that involves bundling data (attributes) and the methods (functions) that manipulate that data into a single unit, the object. This concept restricts direct access to an object's data and allows it to control how data is accessed and modified. Encapsulation promotes data security and helps prevent unintended interference.
Inheritance: Inheritance allows one class (the child or subclass) to inherit attributes and behaviours from another class (the parent or superclass). It promotes code reuse and hierarchical organization. For example, if you have a superclass "Vehicle," you can create subclasses like "Car" and "Motorcycle" that inherit common properties from the parent class while adding their specific features.
Polymorphism: Polymorphism enables objects of different classes to be treated as objects of a common superclass. This concept allows for dynamic method invocation, where different classes can respond to the same method in a way that is appropriate for their specific type. Polymorphism facilitates flexibility in designing and extending software.
Mastering OOP Principles and Methodologies
Abstraction Complexity: Understanding the concept of abstraction, which involves focusing on essential features while ignoring irrelevant details, can be complex for developers new to OOP. It requires a shift in mindset from traditional procedural programming.
Inheritance Hierarchy: Managing complex inheritance hierarchies, especially in large-scale applications, can pose challenges. Developers must carefully plan and design class relationships to avoid potential issues with code maintainability and extensibility.
Encapsulation Implementation: Implementing encapsulation effectively can be difficult, as it involves properly hiding internal data and methods within an object. Without a thorough understanding, developers may inadvertently expose sensitive data, leading to security vulnerabilities.
Polymorphism Application: Applying polymorphism correctly can be challenging, especially when dealing with diverse data types and method invocations. Ensuring that different objects respond appropriately to the same method call requires a solid understanding of class relationships and method overriding.
Conceptual Understanding: Grasping the conceptual shift from procedural to object-oriented thinking can be a hurdle. Developers accustomed to procedural programming may find it challenging to transition to the mindset required for effective OOP design and implementation.
Common Pitfalls in Implementing OOP
Overuse of Inheritance: Inappropriately using extensive inheritance hierarchies can lead to a rigid and overly complex codebase, making it challenging to maintain and extend the application.
Violation of Encapsulation: Failing to enforce proper data hiding can result in data integrity issues and tightly coupled code, hampering the flexibility and reusability of the codebase.
Misunderstanding Polymorphism: Misinterpreting polymorphism may lead to ambiguous or misleading implementations, resulting in unexpected behaviour and potential runtime errors.
Excessive Reliance on Global State: Depending heavily on global variables or states can introduce unintended side effects and make debugging and testing complex, compromising the reliability and predictability of the code.
Lack of Abstraction: Neglecting to implement proper abstraction can lead to code redundancy and reduced maintainability, hindering the scalability and modularity of the software.
How to Become Proficient in Object-Oriented Programming?
To excel in Object-Oriented Programming (OOP), several essential skills are required:
Strong Grasp of Fundamental Programming Concepts: Understanding core programming concepts, such as variables, control structures, and data types, is crucial. Proficiency in essential programming languages is essential for effective OOP development.
Problem-Solving Abilities: OOP often involves solving complex problems. Strong problem-solving skills are vital to break down problems into manageable components and develop effective solutions using OOP principles.
Comprehensive Understanding of Data Structures: OOP heavily relies on data structures for organizing and managing data. A deep understanding of data structures like arrays, linked lists, trees, and graphs is essential for efficient OOP development.
Algorithms Proficiency: Knowledge of algorithms and their efficiency is crucial in OOP. It's essential to select the right algorithms to optimize program performance.
Design Patterns: Familiarity with design patterns like Singleton, Factory, and Observer is valuable for writing clean, maintainable, and scalable code in OOP.
UML (Unified Modeling Language): Understanding UML diagrams for visualizing and communicating OOP designs is beneficial.
Testing and Debugging Skills: Proficiency in testing methodologies and debugging tools is necessary for identifying and resolving issues in OOP code.
Version Control: Knowledge of version control systems (e.g., Git) for collaborative development and code management is important.
Soft Skills: Communication, teamwork, and collaboration skills are vital, as OOP projects often involve working with others.
Continuous Learning: The field of OOP is dynamic, with new technologies and best practices emerging regularly. A commitment to continuous learning is essential to stay up-to-date.
Implementing Object-Oriented Programming in Practice
Define Classes and Objects: Create a class to represent a blueprint for objects, defining attributes and methods. Then instantiate objects based on the defined class.
Encapsulation: Encapsulate data within classes by setting access levels to protect data from unauthorized access. Use access modifiers like public, private, and protected depending on the language.
Inheritance: Implement inheritance by creating a subclass that inherits properties and methods from a parent class. Use inheritance to promote code reusability and establish a hierarchical relationship between classes.
Polymorphism: Implement polymorphism by using method overriding or method overloading. Method overriding allows a subclass to provide a specific implementation of a method that is already provided by its superclass, whereas method overloading allows multiple methods with the same name but different parameters.
Abstraction: Use abstraction to hide complex implementation details and only show the essential features of an object. Abstract classes or interfaces can be used to achieve abstraction.
Implement OOP Design Patterns: Explore and apply common OOP design patterns such as Singleton, Factory, and Observer to solve specific design problems effectively.
Testing and Debugging: Thoroughly test and debug your code to ensure proper functionality and identify and resolve any errors or bugs.
Provide practical advice and effective techniques for writing organized, optimized, and easy-to-maintain code using OOP.
-
Follow SOLID Principles: Embrace SOLID principles (Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) to create modular, reusable, and maintainable code.
-
Use Descriptive Naming Conventions: Employ clear and descriptive names for classes, methods, and variables to enhance code readability and understanding.
-
Encapsulate Properly: Implement encapsulation to restrict access to internal data and ensure data integrity and security within objects.
-
Implement Design Patterns: Apply appropriate design patterns to resolve common design problems and promote efficient code reuse and scalability.
-
Write Unit Tests: Develop comprehensive unit tests to validate the functionality of individual components and ensure the reliability of the codebase.
-
Keep Code Consistent: Maintain consistency in coding style, formatting, and documentation to facilitate collaboration and code maintenance.
-
Avoid Code Duplication: Refactor and reuse code wherever possible to minimize redundancy and promote code simplicity.
-
Use Inheritance Judiciously: Employ inheritance only when necessary to prevent unnecessary code coupling and maintain a clear class hierarchy.
-
Apply Polymorphism: Leverage polymorphism to enable flexibility and extensibility, allowing objects to take on multiple forms and behaviours.
-
Regular Refactoring: Continuously refactor the codebase to improve its structure, performance, and maintainability while adapting to changing requirements.
The Relevance of OOP in Software Development
Object-Oriented Programming (OOP) plays a crucial role in developing scalable, modular, and reusable software applications. By enabling the organization of code into modular objects, OOP promotes the creation of applications that are more adaptable to change and easier to maintain. Through encapsulation, inheritance, and polymorphism, OOP facilitates the building of complex systems with manageable codebases, fostering code reusability and reducing redundancy. Real-world examples such as the development of graphical user interfaces (GUIs), video games, and enterprise-level software solutions demonstrate how OOP principles streamline the development process, enhance code readability, and improve software design. Case studies showcasing the successful application of OOP in building large-scale systems like banking software, content management systems, and e-commerce platforms highlight the advantages of OOP in promoting code extensibility, facilitating collaborative development, and accelerating software deployment, thereby underscoring its pivotal role in modern software development practices.
Key Components and Applications of OOP
Core Components of OOP:
Classes: Classes serve as blueprints for creating objects and defining their properties and behaviours.
Objects: Objects are instances of classes, representing specific entities with their own unique attributes and behaviours.
Methods: Methods are functions within classes that enable objects to perform actions or manipulate data.
Inheritance: Inheritance facilitates the creation of new classes based on existing ones, inheriting their attributes and behaviours.
Advanced OOP Concepts and Practical Applications:
Interfaces: Interfaces define sets of methods that classes must implement, promoting consistency and ensuring certain behaviour across classes.
Abstract Classes: Abstract classes provide a foundation for subclasses and often include abstract methods that must be implemented, facilitating common functionalities among related classes.
Design Patterns: Design patterns offer proven solutions to recurring software design problems, enhancing code structure and maintainability. Common patterns include Singleton, Factory, and Observer, among others.
Object-Oriented Programming (OOP) is a fundamental aspect of modern software development. It promotes the creation of modular, reusable, and efficient code. The core principles of OOP, such as encapsulation, inheritance, and polymorphism, make it easier to develop complex systems with well-organized and manageable codebases. By encouraging the use of classes and objects, OOP allows developers to model real-world entities and their interactions, resulting in software architectures that are more intuitive and easier to maintain.
OOP also plays a crucial role in achieving scalability and code reusability. It empowers programmers to build adaptable solutions that can evolve with changing business requirements. By continuously exploring and implementing OOP principles, developers can enhance their problem-solving skills and cultivate robust software development practices that align with industry standards and best practices.