Introduction to Regular Expressions in Python
Learn about regular expressions, Python, patterns, matching, strings, search, replace, syntax, examples, programming, coding, and text processing.
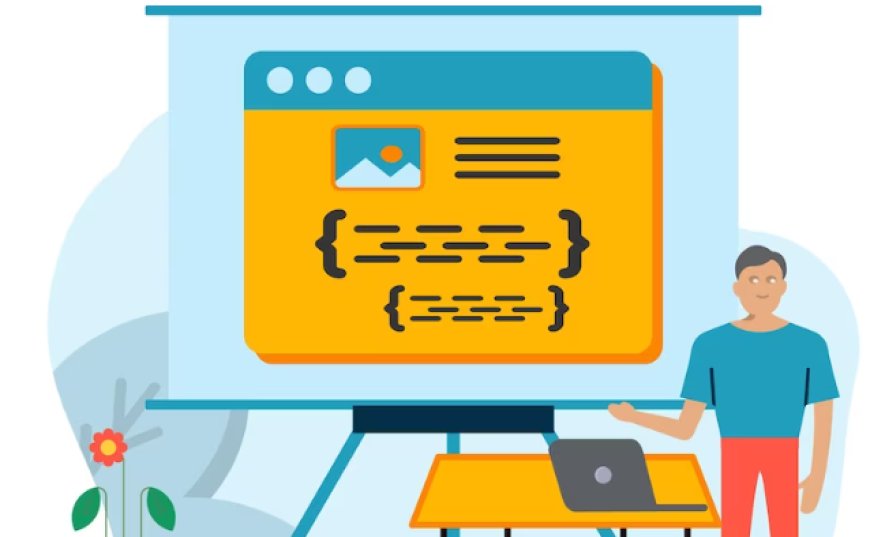
Regular Expressions, often abbreviated as regex or remex, are a powerful tool for pattern matching and manipulation within text. They provide a concise and flexible way to describe complex patterns in strings. A regular expression is essentially a sequence of characters that defines a search pattern. Its primary purpose is to locate and manipulate text based on specific patterns rather than fixed strings. Regular expressions find applications in a wide range of fields, including text processing, data validation, parsing, and search operations. Whether you're validating user inputs, extracting information from large datasets, or performing advanced text transformations, understanding regular expressions can greatly enhance your ability to work with textual data efficiently and effectively.
Basic Syntax of Regular Expressions
-
Raw Strings and Escape Characters
In Python, regular expressions are often written as strings. To avoid conflicts between backslashes in regular expressions and backslashes used in Python strings, it's recommended to use raw strings (indicated by prefixing the string with an 'r'). Raw strings treat backslashes as literal characters, which is crucial for writing regular expressions.
For example, consider the regex pattern `\d{3}\d{2}`, which matches a pattern like "12345". To use this in a raw string, you would write it as `r"\d{3}\d{2}"`.
Escape characters, however, are still used within regular expressions to represent special characters like newline `\n`, tab `\t`, and others. These are similar to escape characters used in regular Python strings but are interpreted within the regex engine.
-
Literal Characters
In a regular expression, most characters are treated as literal characters and match themselves. For instance, if you want to match the string "apple", you can write the regular expression `r"apple"`. The regex engine will try to find occurrences of the exact sequence "apple" in the text.
-
Metacharacters and Their Meanings
Metacharacters are characters that have a special meaning in regular expressions. They allow you to define patterns that are more flexible and powerful.
Here are some common metacharacters and their meanings
`.` (period) Matches any character except a newline.
`^` (caret) Matches the start of a string or the start of a line (with the `re.MULTILINE` flag).
`$` (dollar) Matches the end of a string or the end of a line (with the `re.MULTILINE` flag).
`*` (asterisk) Matches the preceding element zero or more times.
`+` (plus) Matches the preceding element one or more times.
`?` (question mark) Matches the preceding element zero or one time.
`[]` (square brackets) Defines a character class, matching any one character within the brackets.
`()` (parentheses) Groups together expressions and captures the matched text for later use.
`\` (backslash) Escapes a metacharacter, allowing you to match it as a literal character.
These metacharacters enable you to construct complex patterns for matching and searching text efficiently.
Remember that using raw strings is important to ensure that backslashes are interpreted correctly, and understanding the meanings of metacharacters is crucial for building effective regular expressions in Python.
Matching Patterns
Using `re.match()` function
The `re.match()` function is used to match a pattern at the beginning of a string. It tries to match the pattern against the start of the input string. Two important metacharacters used in this context are `^` and `$`.
`^` (caret) This metacharacter asserts the start of a string. It is used to match a pattern only if it appears at the beginning of the input string.
`$` (dollar) This metacharacter asserts the end of a string. It is used to match a pattern only if it appears at the end of the input string.
Example Matching email addresses
```python
import re
pattern = r'^[azAZ09._%+]+@[azAZ09.]+\.[azAZ]{2,}$'
email = "[email protected]"
if re.match(pattern, email)
print("Valid email address")
else
print("Invalid email address")
```
Using `re.search()` function
The `re.search()` function searches for a given pattern throughout the entire input string. It stops at the first match found. It's useful for finding patterns that might not necessarily appear at the start of the string. Grouping and capturing allow you to extract specific parts of the matched text.
-
Finding the first occurrence `re.search()` returns a match object if a match is found, otherwise, it returns `None`.
-
Grouping and capturing Parentheses `( )` are used for grouping parts of the pattern. The text matched by each group can be accessed using `.group()`, `.groups()`, or `.group(index)` methods of the match object.
Using `re.findall()` function
The `re.findall()` function is used to find all occurrences of a pattern in a string. It returns a list of all matched substrings.
-
Finding all occurrences `re.findall()` returns a list of all non overlapping matches.
-
Non Capturing groups You can use `(? ... )` to create non-capturing groups. These groups are used for grouping, but they don't store the matched text as a separate group.
Character Classes and Quantifiers
Character classes allow you to specify a set of characters that you want to match. They are enclosed in square brackets `[ ]` and can include individual characters, ranges of characters, or predefined shorthand character classes.
Individual CharactersYou can list specific characters you want to match, like `[abc]` which will match any occurrence of 'a', 'b', or 'c'.
Character RangesTo match a range of characters, you can use a hyphen `-` between the start and end characters. For example, `[a-z]` matches any lowercase letter from 'a' to 'z'.
Predefined Shorthand Classes
`\d` Matches any digit (equivalent to `[0-9]`).
`\w` Matches any word character (alphanumeric character plus underscore).
`\s` Matches any whitespace character (spaces, tabs, newlines).
`\D`, `\W`, `\S` Negations of `\d`, `\w`, and `\s`, respectively.
Quantifiers
Quantifiers determine how many times a preceding element (character or group) should occur in the text for a match to be found.
`*` (asterisk) Matches zero or more occurrences of the preceding element. For example, `a*` matches zero or more 'a' characters.
`+` (plus) Matches one or more occurrences of the preceding element. For example, `a+` matches one or more 'a' characters.
`?` (question mark) Matches zero or one occurrence of the preceding element. For example, `colou?r` matches both "color" and "colour".
Advanced Pattern Matching
Advanced pattern matching in regular expressions involves more complex techniques for refining and customizing the way patterns are matched within a given text. These techniques allow you to express more sophisticated conditions that patterns must satisfy. Here are some key concepts within advanced pattern matching
-
Alternation with `|` Alternation allows you to specify multiple alternative patterns, separated by the pipe symbol `|`. The regex engine will try to match any of the alternatives. For example, `(cat|dog)` will match either "cat" or "dog".
-
Grouping and Backreferences Parentheses `()` are used to create groups within your pattern. These groups can then be referenced later in the pattern using backreferences (`\1`, `\2`, etc.). This is useful when you want to match repeated occurrences of the same substring, like in patterns that involve repeating words.
-
Lookahead and Lookbehind Assertions Lookahead and lookbehind assertions allow you to specify conditions that must be true either ahead or behind the current position, without actually consuming any characters. Positive lookahead assertion is written as `(?=...)`, while negative lookahead is written as `(?!...)`. Similarly, positive lookbehind is written as `(?<=...)`, and negative lookbehind is written as `(?
Example Matching Phone Numbers with Specific Formats
Let's say you want to match phone numbers in the formats "xxx-xxx-xxxx" or "(xxx) xxx-xxxx". You can use alternation and grouping to achieve this
```
(\d{3}-\d{3}-\d{4})|(\(\d{3}\) \d{3}-\d{4})
```
In this pattern
- `(\d{3}-\d{3}-\d{4})` matches the xxx-xxx-xxxx format.
- `(\(\d{3}\) \d{3}-\d{4})` matches the (xxx) xxx-xxxx format.
Regular expressions (regex) in Python are a powerful tool for pattern matching and manipulation within strings. They provide a concise and flexible way to search, extract, and replace text based on specific patterns. Python's built-in `re` module enables users to work with regular expressions efficiently, offering functions to match patterns, find all occurrences, substitute text, and split strings. While regular expressions can be incredibly useful, they can also become complex and hard to read for intricate patterns. As such, it's recommended to strike a balance between utilizing regular expressions effectively and maintaining code readability.