Exception Handling in Software Development
Explore exception handling, software development, errors, bugs, coding, best practices, strategies, programming, debugging, solutions, and resilience.
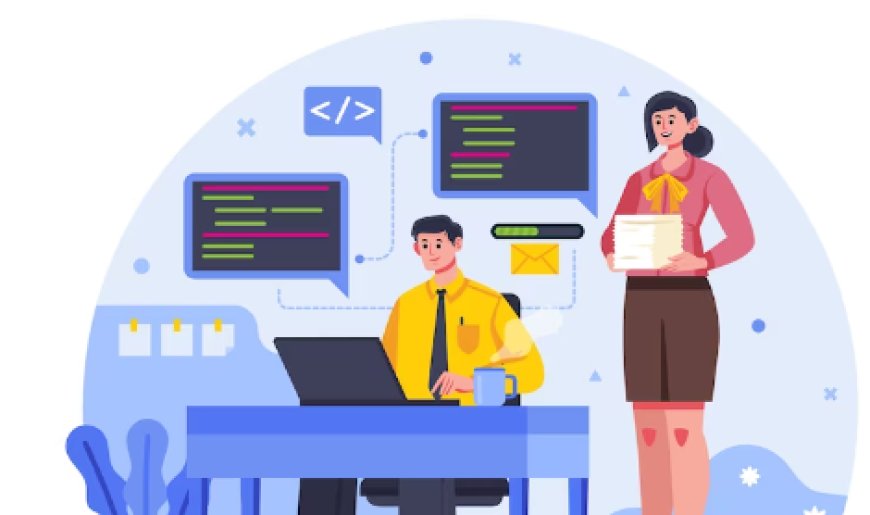
In the world of programming, unexpected events and errors are inevitable. Exception handling in Python is a crucial mechanism that allows developers to gracefully manage these unforeseen situations, ensuring that programs continue to function even when errors occur. An exception is an event that disrupts the normal flow of a program, and Python's exception handling mechanism enables developers to catch, analyze, and respond to these events. By using try except blocks, programmers can anticipate potential issues, handle them appropriately, and maintain the overall robustness and reliability of their code. In this section, we will delve into the fundamentals of exception handling, exploring its significance and the foundational components that enable developers to create more resilient applications.
Basic Exception Handling
Exception handling is a crucial concept in programming that allows you to manage and handle errors that might occur during the execution of your code. It helps prevent your program from crashing when unexpected situations arise and provides a way to gracefully handle those situations.
Basic exception handling involves the following key
components
-
Try The code that might raise an exception is placed within a `try` block. This is the portion of the code where you anticipate potential errors.
-
ExceptThe `except` block follows the `try` block and contains the code that should be executed when an exception occurs within the associated `try` block. You specify the type of exception you want to catch after the `except` keyword.
-
RaiseYou can intentionally raise exceptions using the `raise` keyword. This is useful when you want to trigger a specific exception based on a certain condition in your code.
Here's a basic example in Python to illustrate exception handling
```python
try
num = int(input("Enter a number "))
result = 10 / num
print("Result", result)
except ZeroDivisionError
print("Cannot divide by zero.")
except ValueError
print("Invalid input. Please enter a valid number.")
except Exception as e
print("An error occurred", e)
else
24082023
01
print("No exceptions occurred.")
finally
print("Exception handling is complete.")
```
In this example
-
The `try` block contains code that accepts user input, performs a division, and prints the result.
-
The `except` blocks handle specific exceptions, such as `ZeroDivisionError` and `ValueError`, providing appropriate error messages.
-
The `else` block is executed if no exceptions are raised within the `try` block.
-
The `finally` block contains code that will be executed regardless of whether an exception occurred or not. It's commonly used for cleanup tasks.
It's important to catch specific exceptions that you anticipate might occur while leaving more general exceptions (like `Exception` in the example) as a catchall for unexpected situations. This helps you provide tailored error messages and maintain the program's stability.
Basic exception handling improves the robustness of your code and provides a way to guide the program's behavior even when things go wrong.
Exception Hierarchy and Custom Exceptions
-
Exception Hierarchy
In programming, exceptions are events that occur during the execution of a program that disrupts the normal flow of the program's instructions. Exception handling is a technique used to gracefully manage these unexpected situations, allowing the program to handle errors and continue running rather than crashing.
An exception hierarchy refers to the organization of different types of exceptions in a structured manner. In many programming languages, including Java and Python, exceptions are organized into a hierarchy or treelike structure. At the root of this hierarchy, you typically have a base or generic exception class that captures the most general types of errors. This base class is usually inherited by more specific exception classes that represent different categories of errors.
For example, in Java, you have the `Throwable` class at the root of the hierarchy, which is further divided into `Error` and `Exception`. The `Exception` class is then subclassed into more specific exceptions like `IOException`, `NullPointerException`, and so on. This hierarchy allows programmers to catch and handle different types of exceptions at different levels, providing a more granular control over error handling.
-
Custom Exceptions
While programming languages provide a standard set of exceptions to cover common error scenarios, you can also create your own exceptions, known as custom exceptions. Custom exceptions allow you to define specific error conditions that are relevant to your application or domain. This can make your code more readable, maintainable, and tailored to your application's needs.
To create a custom exception, you typically define a new class that inherits from a base exception class (such as `Exception` in Python or a similar base class in other languages). You can then add additional properties and methods to your custom exception class to provide more information about the error and potentially even define custom behavior when the exception is raised and caught.
Custom exceptions are particularly useful when you're building larger applications, libraries, or frameworks, as they allow you to establish a more meaningful and context specific way of handling errors. By creating a well organized exception hierarchy and using custom exceptions where appropriate, you can make your code more robust and easier to debug.
Handling Exceptions in Practical Scenarios
Handling exceptions in practical scenarios is a fundamental concept in programming and software development. Exceptions are unexpected events or errors that can occur during the execution of a program, disrupting its normal flow. These events can range from simple issues like dividing by zero to more complex problems like file I/O errors or network connectivity problems. Effectively handling exceptions is crucial to ensure that your program remains stable, userfriendly, and responsive in the face of unexpected situations.
-
TryCatch Blocks
The primary mechanism for handling exceptions is using try catch blocks. In many programming languages, you enclose the code that might cause an exception within a `try` block. If an exception occurs within the `try` block, the program jumps to the corresponding `catch` block, which contains code to handle the exception gracefully.
-
Exception Types
Exceptions are often categorized into different types based on the nature of the problem. Common exception types include division by zero, null pointer reference, file not found, input/output errors, and network related issues.
-
Catching Specific Exceptions
You can catch specific exceptions by specifying the exception type in the `catch` block. This allows you to tailor your errorhandling code to different scenarios. For instance, if you're dealing with file operations, you might catch `FileNotFoundException` separately from other types of exceptions.
-
Exception Handling Strategies
Logging Logging exceptions is essential for debugging and maintenance. When an exception occurs, log relevant information such as the error message, timestamp, and the state of the program at that point. This information can help you diagnose issues and improve the robustness of your program.
UserFriendly Messages When an exception occurs, displaying cryptic error messages to users can be frustrating and unhelpful. Instead, provide clear and understandable error messages that guide users on what went wrong and how they can address it.
Advanced Exception Handling Techniques
Advanced exception handling techniques are strategies and methodologies employed in software development to effectively manage and respond to unexpected or exceptional situations that can arise during the execution of a program. While traditional exception handling primarily involves using try catch blocks to handle known exceptions, advanced techniques delve deeper into addressing complex scenarios and enhancing the overall reliability and maintainability of software systems.
One advanced technique is "Exception Chaining," which involves capturing an exception, appending additional contextual information, and rethrowing it. This aids in providing comprehensive error information to aid debugging and troubleshooting. Another technique is "Custom Exception Classes," where developers create their own exception classes that inherit from the standard exception classes provided by the programming language. This enables better categorization and differentiation of exceptions based on application specific logic.
"Exception Logging" is another crucial technique that involves recording exception details, such as the stack trace, error messages, and relevant input data, into log files. This facilitates postmortem analysis of errors and helps developers identify the root causes of issues more efficiently.
"Graceful Degradation" is an advanced strategy often employed in systems that require high availability. It involves anticipating potential failures and designing the software to continue functioning with reduced functionality, rather than crashing completely. This approach is common in distributed systems and web applications where maintaining a seamless user experience is paramount.
Exception handling in Python is a vital programming concept that enables developers to gracefully manage and respond to errors or unexpected events during program execution. By using try-except blocks, developers can isolate risky code and define specific actions to take when exceptions occur. Python's rich set of builting exceptions and the ability to create custom exceptions offer flexibility in handling various scenarios. A well designed exception handling strategy enhances code reliability, user experience, and overall program robustness by preventing crashes and providing informative error messages.