Debugging and Testing in Python
Master Python debugging, testing, errors, unittests, pytest, code quality, breakpoints, automation, efficiency, and solutions. Enhance code reliability
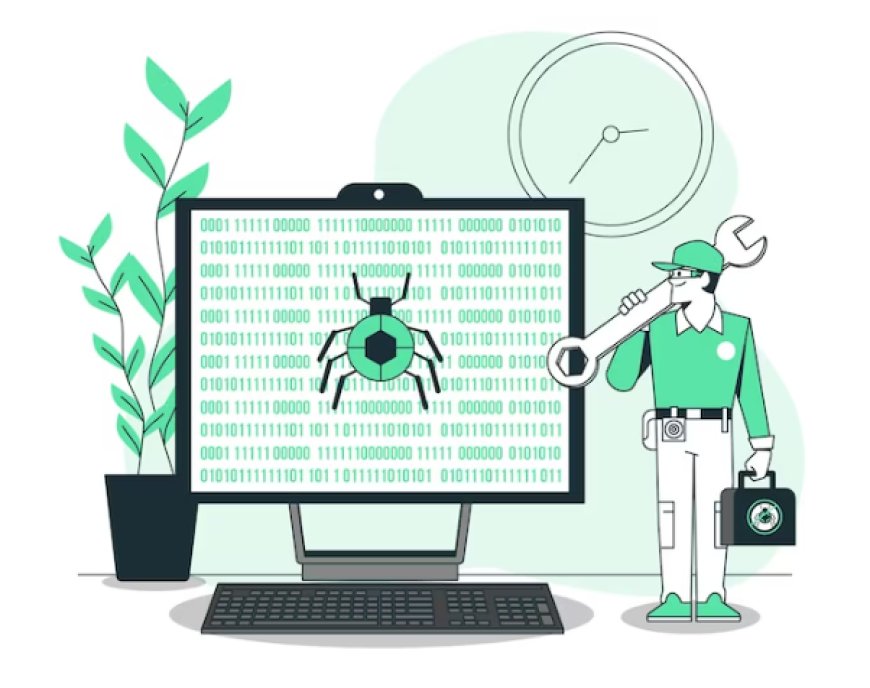
Debugging and testing are crucial components of the software development process. In Python, these practices play a vital role in ensuring the reliability, functionality, and quality of your code. In this blog, we will delve into the world of debugging and testing in Python, exploring effective strategies, tools, and techniques to identify and fix bugs, as well as validate the correctness of your code through comprehensive testing.
Understanding Debugging
Debugging is the process of identifying and resolving issues, or bugs, within your code. These bugs can cause unexpected behaviors, crashes, or incorrect results. Python provides powerful debugging tools and techniques that help developers trace and analyze code execution, isolate problems, and rectify errors efficiently.
Printing and Debugging Statements
One of the simplest and most effective debugging techniques is using print statements to display variable values, control flow, and intermediate results during program execution. By strategically placing print statements at critical points in your code, you can gain insights into its behavior and identify potential issues.
Debugging with pdb:
Python's built-in debugger, pdb, provides a more advanced approach to debugging. It allows you to set breakpoints, step through code execution, inspect variables, and analyze stack traces. By utilizing pdb, you can interactively debug your code, gaining a deeper understanding of its behavior and resolving complex issues.
Logging:
Logging is another powerful debugging technique that allows you to capture and record runtime information for analysis. The logging module in Python provides a flexible and configurable logging framework, enabling you to log messages with various levels of severity. By strategically logging relevant information, you can track the execution flow and pinpoint problematic areas in your code.
Effective Testing in Python
Testing is a critical process in software development that aims to validate the correctness and reliability of your code. Python offers a variety of testing frameworks and tools that simplify the testing process and promote good testing practices.
Unit Testing with unittest:
The unittest module in Python provides a comprehensive framework for writing and running unit tests. Unit tests focus on testing individual units of code, such as functions or methods, in isolation. By creating unit tests, you can verify the behavior and correctness of specific code units, ensuring they produce the expected results.
Test Driven Development (TDD):
Test Driven Development is an iterative development process that involves writing tests before writing the actual code. With TDD, you start by creating a test that describes the desired functionality, run the test (which fails initially), and then write the code to make the test pass. This approach promotes better code design, modularity, and test coverage.
Integration and System Testing:
In addition to unit tests, integration and system tests are crucial for verifying the interactions and behavior of components within a larger system. Integration tests focus on testing the integration points between different modules or components, while system tests verify the functionality and behavior of the complete system as a whole.
Test Coverage Analysis:
Test coverage analysis measures the extent to which your tests cover the codebase. It helps identify areas of your code that are not adequately tested, highlighting potential gaps in test coverage. By analyzing test coverage metrics, you can ensure that critical sections of your code are thoroughly tested, reducing the risk of undetected bugs.
Test Automation:
Automating your tests using frameworks like pytest or nose can significantly streamline the testing process. Automation allows you to run tests in a repeatable and consistent manner, saving time and effort. Additionally, it facilitates continuous integration and deployment practices, ensuring that your code remains reliable and bug-free throughout the development cycle.
Expanding on Debugging and Testing Techniques
Exception Handling and Error Reporting: Proper exception handling is essential for robust code. By catching and handling exceptions gracefully, you can prevent crashes and provide informative error messages to users. Use try-except blocks to capture specific exceptions and handle them appropriately. Additionally, consider implementing error reporting mechanisms to collect and analyze error information in production environments, allowing you to address issues proactively.
Debugging with IDEs and Debugging Tools: Integrated Development Environments (IDEs) offer powerful debugging features that enhance your debugging capabilities. IDEs like PyCharm, Visual Studio Code, and PyDev provide intuitive debugging interfaces, breakpoints, variable inspection, and step-by-step execution. Leveraging these tools can significantly streamline the debugging process and improve your efficiency as a developer.
Debugging Techniques for Specific Scenarios
Remote Debugging: Sometimes, bugs only surface in specific environments or scenarios. Remote debugging allows you to connect to a running program remotely and analyze its behavior. This technique is especially useful for debugging server-side applications or distributed systems.
Debugging Web Applications: For web development, browser developer tools and frameworks like Django Debug Toolbar provide insights into HTTP requests, database queries, and template rendering. Utilizing these tools enables efficient debugging of web applications.
Debugging Concurrent Code: Debugging multi-threaded or asynchronous code can be challenging due to race conditions and timing issues. Techniques like logging, synchronization primitives, and thread-safe debugging tools (e.g., pdb++, PyCharm's concurrency debugger) can help you diagnose and resolve such issues.
Test Data Generation and Mocking: To ensure comprehensive testing, it's crucial to have diverse and representative test data. Test data generation tools like Faker or Hypothesis can help generate realistic and randomized data for testing. Additionally, mocking frameworks like unittest.mock or pytest-mock allow you to simulate external dependencies or complex interactions, enabling focused and isolated testing.
Continuous Integration and Test Suites: Integrating testing into your development workflow through continuous integration (CI) is essential. CI tools like Jenkins, Travis CI, or GitHub Actions can automatically run your test suite whenever code changes are pushed, ensuring that regressions or errors are caught early. This practice promotes a stable codebase and helps maintain code quality throughout the development process.
Regression Testing and Test Maintenance: As your codebase evolves, it's crucial to update and maintain your test suite. Regression testing involves re-running tests to ensure that modifications or new features haven't introduced unexpected bugs. Regularly reviewing and updating tests, especially after code refactoring or significant changes, helps maintain the reliability of your test suite.
Debugging and testing are essential practices in Python programming that help you build reliable, robust, and bug-free code. By utilizing effective debugging techniques, such as print statements, debugging tools like pdb, and logging, you can identify and resolve issues efficiently.
As you continue your Python programming journey, invest time in mastering debugging and testing techniques. Embrace good debugging practices, write comprehensive tests, and leverage the available tools and frameworks to ensure the stability and quality of your code.
By incorporating debugging and testing into your development process, you'll be equipped to create reliable, bug-free applications that provide an excellent user experience and stand up to real-world scenarios.